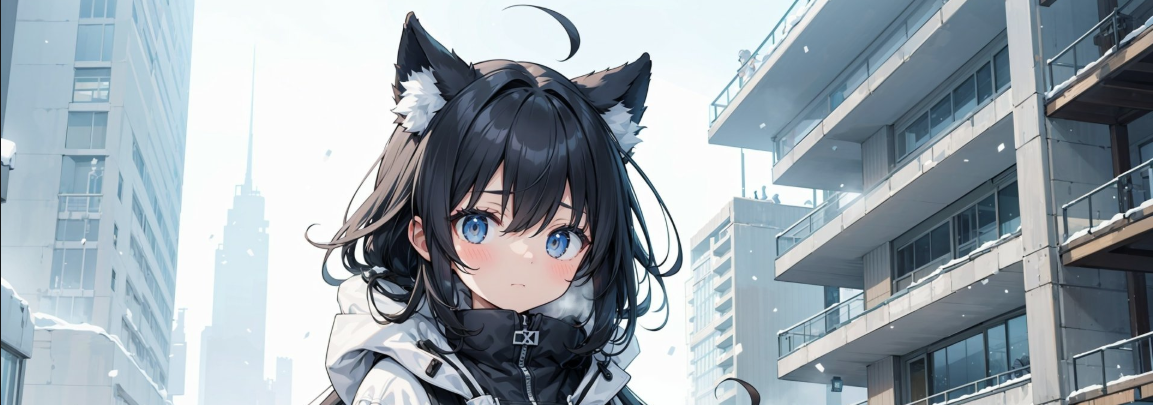
Maya 脚本 帮助
2024年12月8日
本文介绍Maya中常用的脚本帮助。
常规情况下 我们是会通过 下方连接两个不同的api帮助来查询Maya的命令。
- https://help.autodesk.com/view/MAYAUL/2024/CHS/index.html?contextId=COMMANDS-INDEX (Maya Command)
- https://help.autodesk.com/view/MAYAUL/2024/CHS/index.html?contextId=COMMANDSPYTHON-INDEX (Maya Python Command)
还有一种情况就是我们得对属性编辑器里的节点属性进行查询,这时候我们就可以通过
- https://help.autodesk.com/view/MAYAUL/2024/CHS/index.html?contextId=NODES-INDEX(Maya Node)
这两者结合起来 我们就可以查询到大部分的Maya命令和属性。
查询和设置节点属性
在Maya中,可以使用Python脚本来查询和设置节点的属性。以下是一些示例代码:
查询节点的所有属性
import maya.cmds as cmds
# 假设你有一个名为 'creaseSet1' 的节点
node_name = 'creaseSet1'
# 列出节点的所有属性
attributes = cmds.listAttr(node_name)
print(f"节点 '{node_name}' 的属性列表:")
for attr in attributes:
print(attr)
查询特定属性的详细信息
import maya.cmds as cmds
# 查询 'creaseLevel' 属性的当前值
crease_level = cmds.getAttr('creaseSet1.creaseLevel')
print(f"当前的 creaseLevel 值: {crease_level}")
设置属性值
import maya.cmds as cmds
# 设置 'creaseLevel' 属性的新值
new_level = 2.0
cmds.setAttr('creaseSet1.creaseLevel', new_level)
print(f"新的 creaseLevel 值: {new_level}")
处理不同类型的属性
在Maya中,有些属性可能是复合属性、数组属性,或者是没有直接值的属性。以下是一些处理这些情况的方法:
检查属性是否存在
import maya.cmds as cmds
# 检查属性是否存在
node_name = 'creaseSet1'
attr_name = 'memberWireframeColor'
if cmds.attributeQuery(attr_name, node=node_name, exists=True):
try:
attr_value = cmds.getAttr(f'{node_name}.{attr_name}')
print(f"当前的 {attr_name} 值: {attr_value}")
except Exception as e:
print(f"无法获取属性值: {e}")
else:
print(f"属性 '{attr_name}' 不存在于节点 '{node_name}' 中。")
处理复合属性和数组属性
import maya.cmds as cmds
# 假设 'memberWireframeColor' 是一个复合属性
compound_attr = 'creaseSet1.memberWireframeColor'
# 获取复合属性的子属性
child_attrs = cmds.attributeQuery('memberWireframeColor', node='creaseSet1', listChildren=True)
print(f"复合属性的子属性: {child_attrs}")
# 获取数组属性的值
# 假设 'someArrayAttr' 是一个数组属性
array_attr = 'creaseSet1.someArrayAttr'
if cmds.attributeQuery('someArrayAttr', node='creaseSet1', multi=True):
array_values = cmds.getAttr(array_attr)
print(f"数组属性的值: {array_values}")
处理连接属性
import maya.cmds as cmds
# 检查属性是否有连接
connections = cmds.listConnections(f'{node_name}.{attr_name}', plugs=True)
if connections:
print(f"属性 '{attr_name}' 连接到: {connections}")
else:
print(f"属性 '{attr_name}' 没有连接。")
通过这些方法,你可以更好地处理和判断Maya中不同类型的属性。